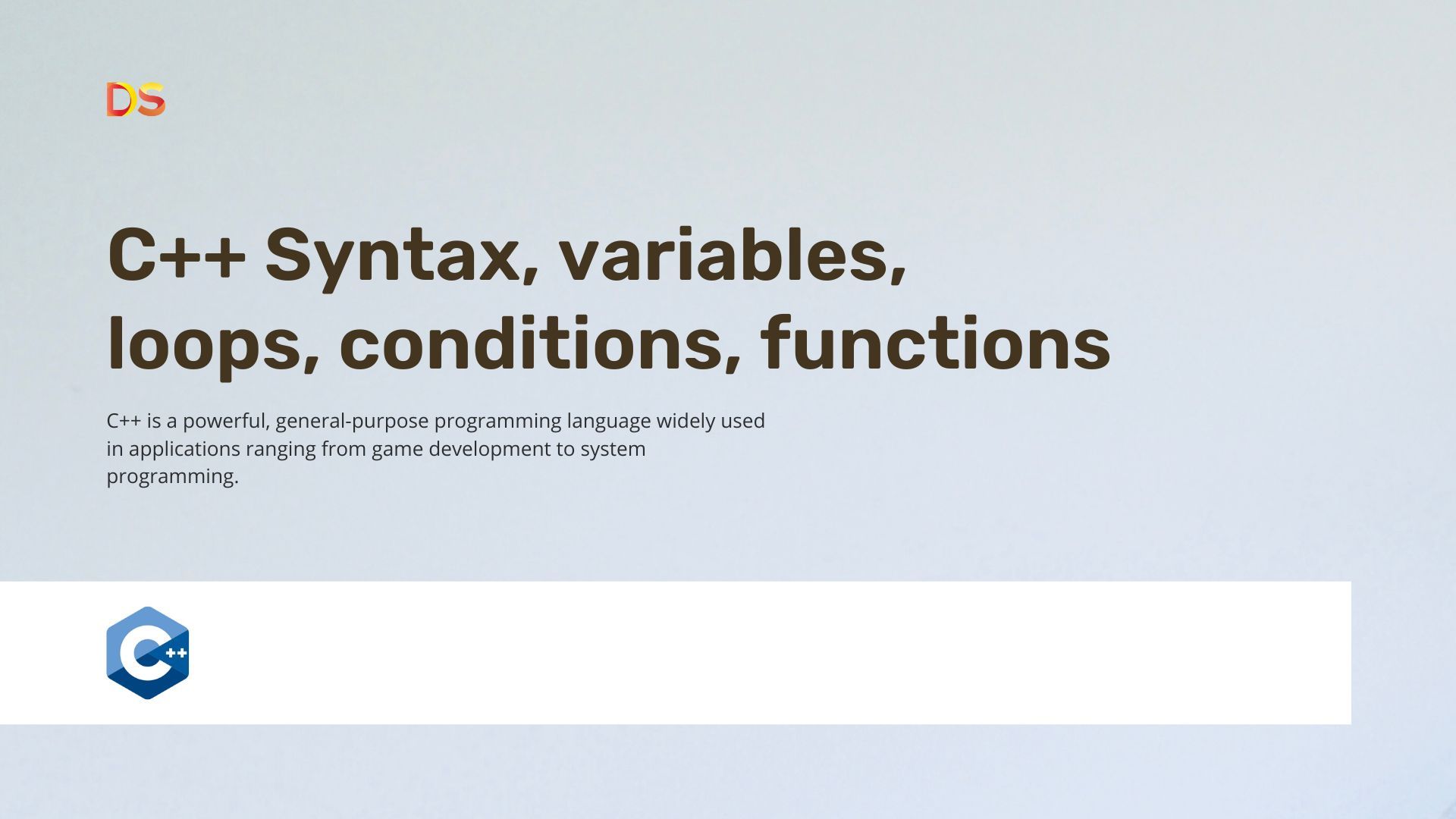
C++ is a powerful, general-purpose programming language widely used in applications ranging from game development to system programming. For beginners, mastering its fundamental concepts - syntax, variables, loops, conditions and functions - is essential to building a strong programming foundation.
C++ is an extension to the existing C language and adds the following:
- Creation and using of abstract data types
- Object Oriented Programming
- Improvements of C like types, inheritance, polymorphism.
In this article, I am going to explore these core topics, offering explanations, code examples and some practices to help learners understand and apply C++.
C++ Syntax
Every C++ program follows a structured format and some key elements. It has at least one function, one of which must be main(). The operating system runs the program through calling this function.
A function itself has four elements: a name, arguments, a body and a return type.
Let’s examine the code.
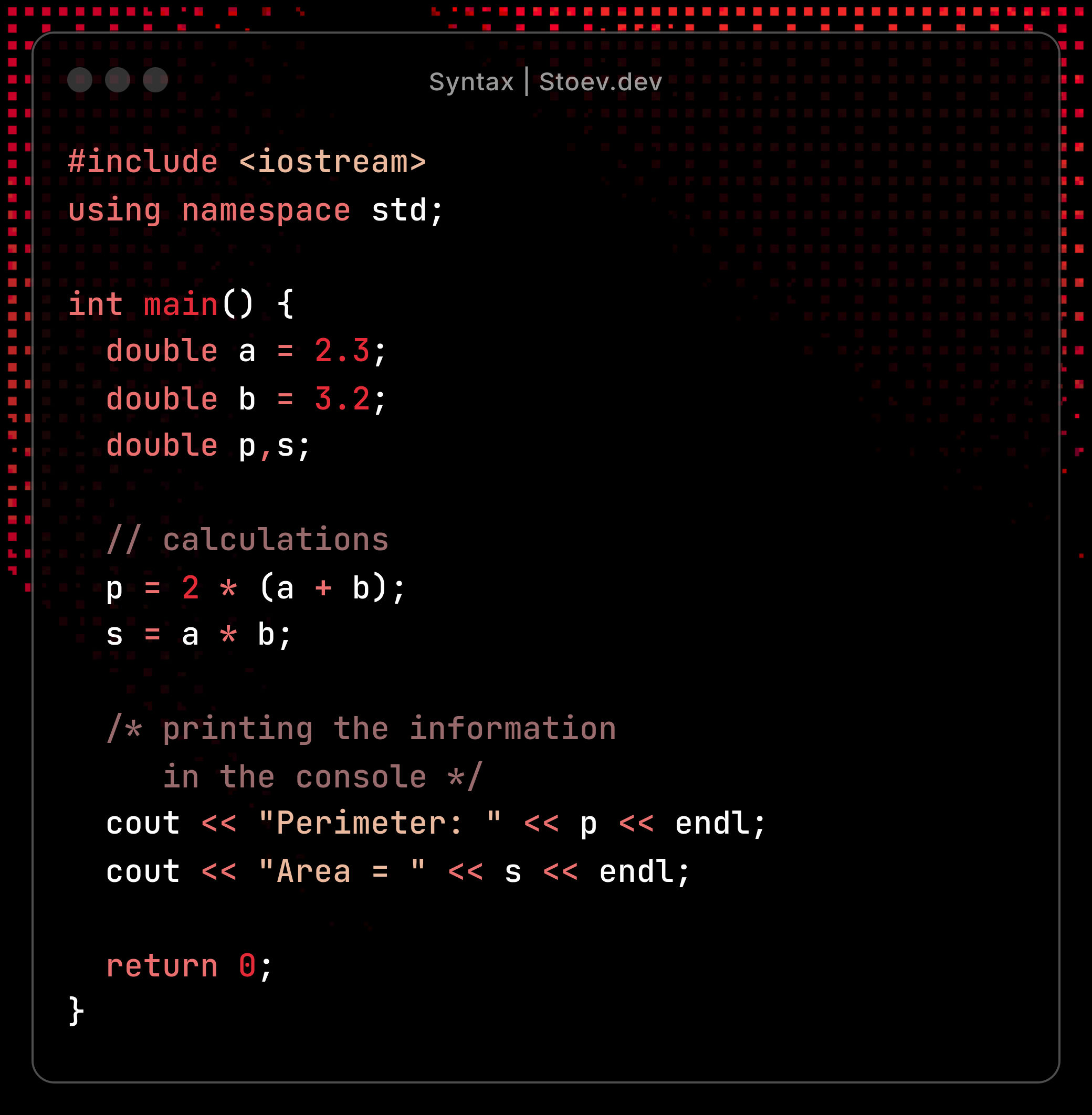
- Preprocessor Directives - Lines starting with #, like #include<iostream> import libraries for functionality such as input/output. Also called a header.
- Namespace - The std namespace contains standard library functions. It makes the names of the std visible.
- Main() - This is the starting entry point where execution begins.
- Declaration of variables - In the body we start to write the functionality of our application. We do basic calculations.
- << - This is an insertion operator, used to send data to the cout - an object of std::ostream that outputs data to the console.
- endl - A manipulator that inserts a newline character \n and flushes the output buffer, ensuring immediate display. Flushing forces the buffered output to be written to the console, which is useful for debugging. If a program crashes, and the output is not flushed, it may lead to incorrect inferences about where the program crashed.
- Comments - We can have single lines comments and multiline comments as well.
- Semicolons - Terminate statements, like a period in a sentence. Extremely important in C++.
- return 0 - Signals successful program completion to the operating system.
Variables and Data Types
Variables store data for processing. Declaring a variable is simple with the syntax: type variableName; C++ is statically typed, meaning the type must be known at compile time and cannot change.
Variable names are case sensitive. That would mean that Age ≠ age. They also have scope (local within a function or global across the program). Local variables must be initialized before use to avoid undefined behavior. When defining a variable, we can also not state its type, but we can define it as auto. The type is deduced from the initializer.
Variables are allocated in memory based on their type’s size, typically on the stack for local variables. The exact address can be accessed using the & operator (e.g. &age).
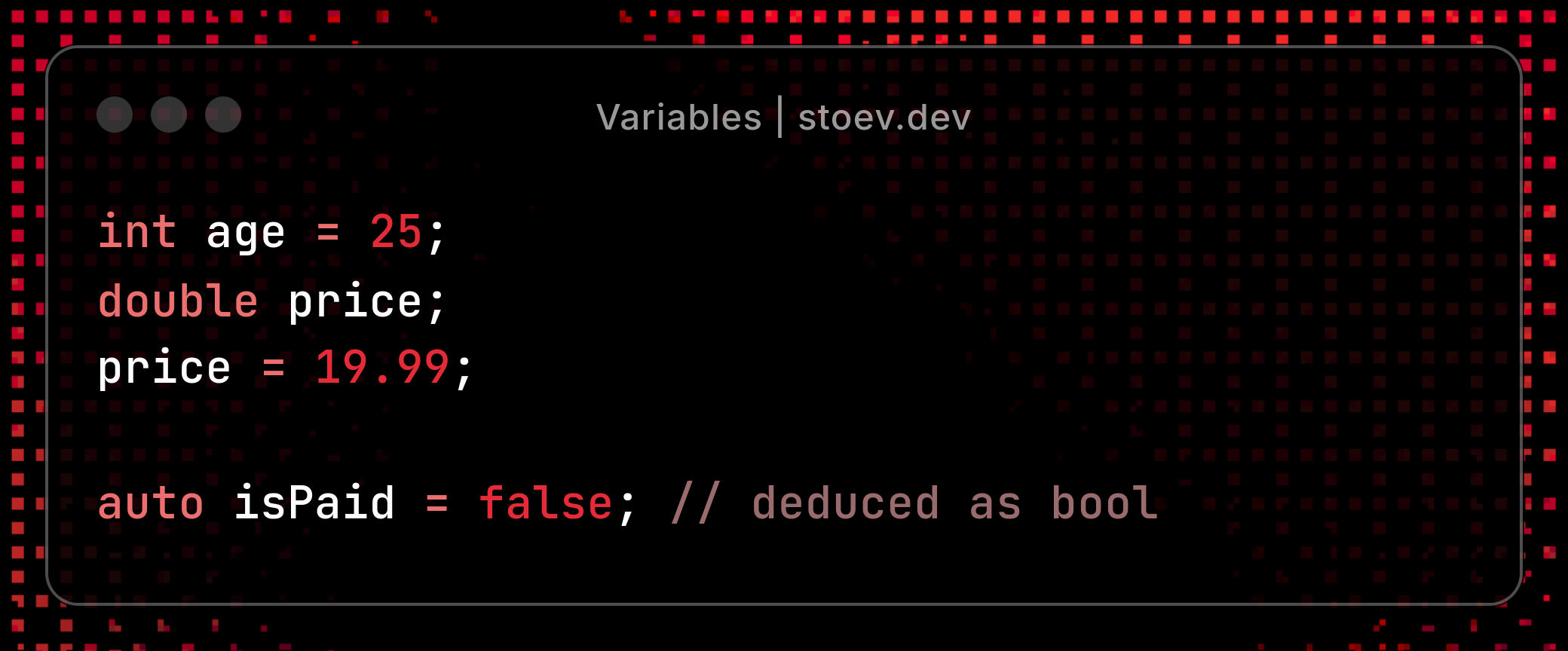
Here are all the data types in C++:
Numeric Types
- int: 2 or 4 bytes, with a range of -2^31 to 2^31-1 (-2,147,483,648 to 2,147,483,647) for signed.
- unsigned int: The same size, but the range is 0 to 2^32-1 (0 to 4,294,967,295).
- short: 2 bytes, signed range -32,768 to 32,767.
- unsigned short: 0 to 65,535.
- long: At least 4 bytes, often same as int on 32-bit systems, but may be 8 bytes on 64-bit systems. Signed range typically -2^31 to 2^31-1 or larger.
- unsigned long: Non-negative, up to 2^21-1 or higher.
- long long: (yes that is a real type) - At least 8 bytes, signed range -2^63 to 2^63-1 (-9,223,372,036,854,775,808 to 9,223,372,036,854,775,807).
- unsigned long long: 0 to 2^64-1.Floating-point types store numbers with decimal points, following the IEEE 754 standard for precision.
- float: 4 bytes, with 6 significant digits of precision. Range: ~1.18e-38 to ~3.4e38.
- double: 8 bytes, with up to 15 decimal digits of precision. Range: ~2.23e-308 to ~1.8e308.
- long double: Often 10-16 bytes, platform-dependent, with higher precision (e.g. 18-19 digits on some systems).
> Precision: double is preferred for most scientific calculations due to its balance of precision and performance. float is often used in memory-constrained environments (e.g. graphics). long double is less common, but useful for extreme precisions.
> Signed vs Unsigned: Signed types use one bit for the sign (positive/negative), halving the positive range compared to unsigned. Unsigned types are ideal for quantities that are inherently non-negative. Mixing calculation of signed and unsigned can yield surprising results when the signed value is negative. Signed values are automatically converted to unsigned.
Character Types
- char: 1 byte. Range: -128 to 127 (signed) or 0 to 255 (unsigned). Stores ASCII characters (e.g., 'A' = 65). Computations using char are problematic, because char is signed on some machines, while not on others. Specify!
- wchar_t: Wide character for Unicode or extended character sets (e.g. L’A’). Size is platform dependent.
- char16_t: Exactly 2 bytes, for UTF-16 encoding.
- char32_t: Exactly 4 bytes, for UTF-32 encoding.
Boolean Type
- bool: Stores true or false, typically 1 byte is needed.
String Type
- String: Not a built-in type, but provided by the <string> library. Stores a sequence of char for text. Supports operations like concatenation and length checking.
Constants
Constants are variables whose values cannot be modified after initialization.
const double PI = 3.14.159;
- const: Prevents modification, evaluated at runtime or compile time depending on context.
- constexpr: Variables declared as such are implicitly const and must be initialized by constant expressions.
I am going to mention the extern keyword here. It is used to declare a variable or a function whose definition is present in some other file. Basically it extends the visibility of the variables and function to multiple source files. It specifies that the symbol has external linkage.
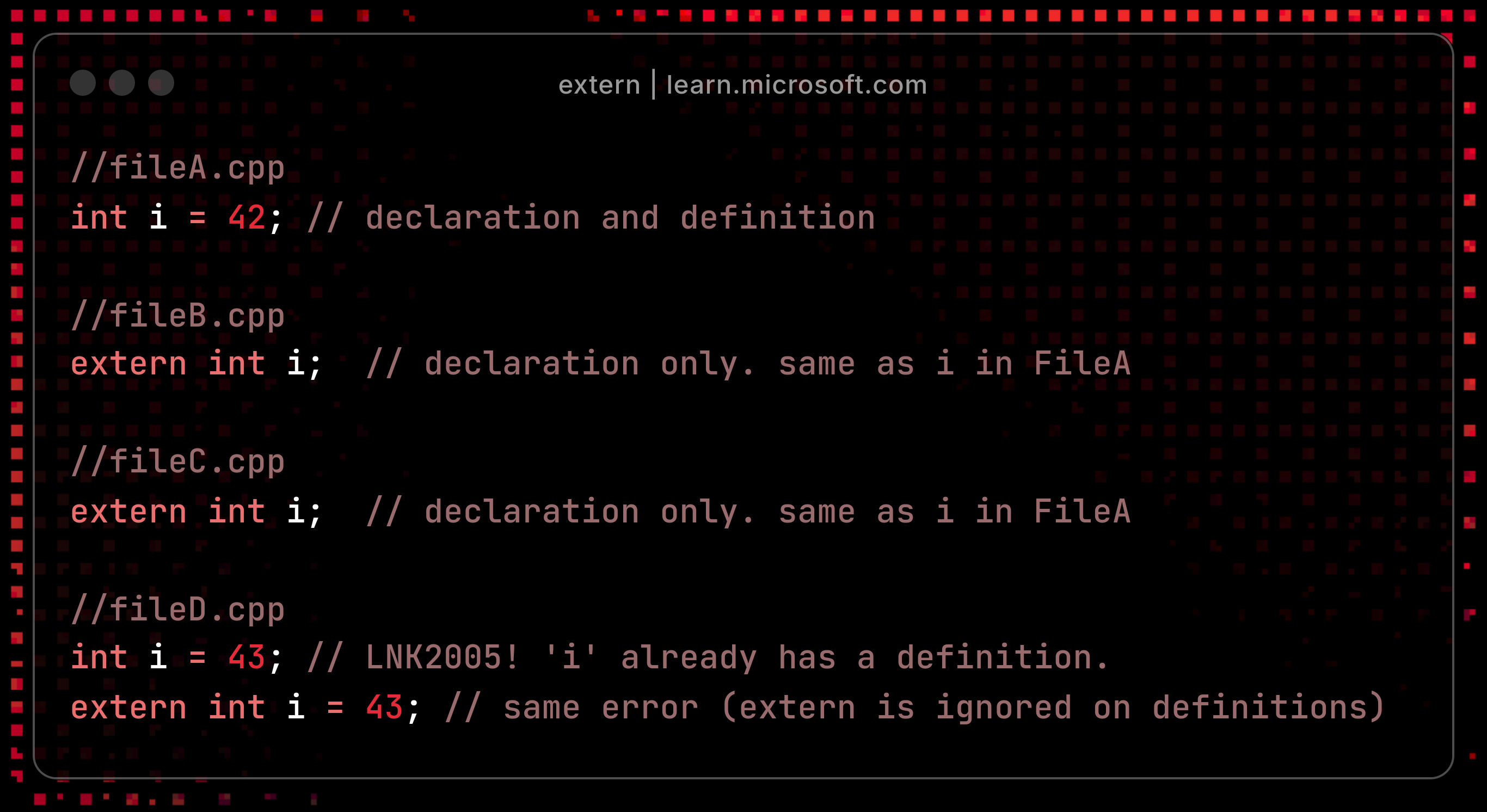
Loops
Loops enable repetitive execution of code, critical for tasks like iteration or event handling. C++ provides three loop constructs, each with specific use cases and behaviors.
A block of code is executed while a condition holds or for a specified number of iterations. They rely on:
- Initialization: Setting up loop variables.
- Condition: Determining whether to continue.
- Update: Modifying variables to eventually terminate the loop.
Improper loop design can lead to infinite loops or off-by-one errors, so careful condition crafting is essential.
For Loop
The for loop is suited for iterations with a known count.
- Initialization: Executes once (e.g. int i = 0).
- Condition: Checked before each iteration (e.g. i < n).
- Update: Executes after each iteration (e.g. I++).
We also have a range-based loop for anything that is iterable, such as arrays and strings. It provides a more readable and concise syntax.
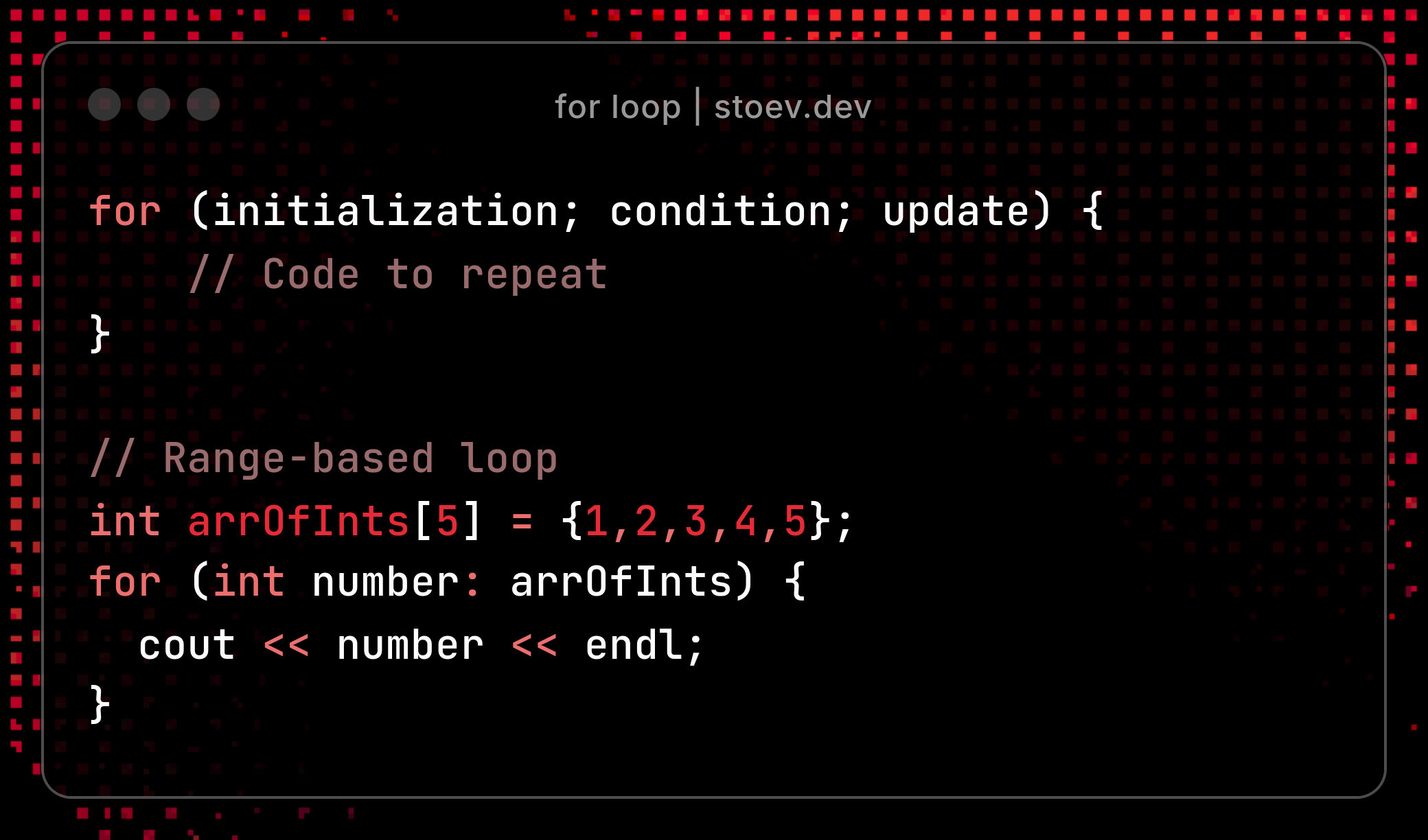
While loop
The while loop runs as long as a condition is true, ideal for indeterminate iterations. The syntax is simple: while (condition) -> do something. Also worth mentioning - when you create variables inside the body, they are created and destroyed on each iteration.
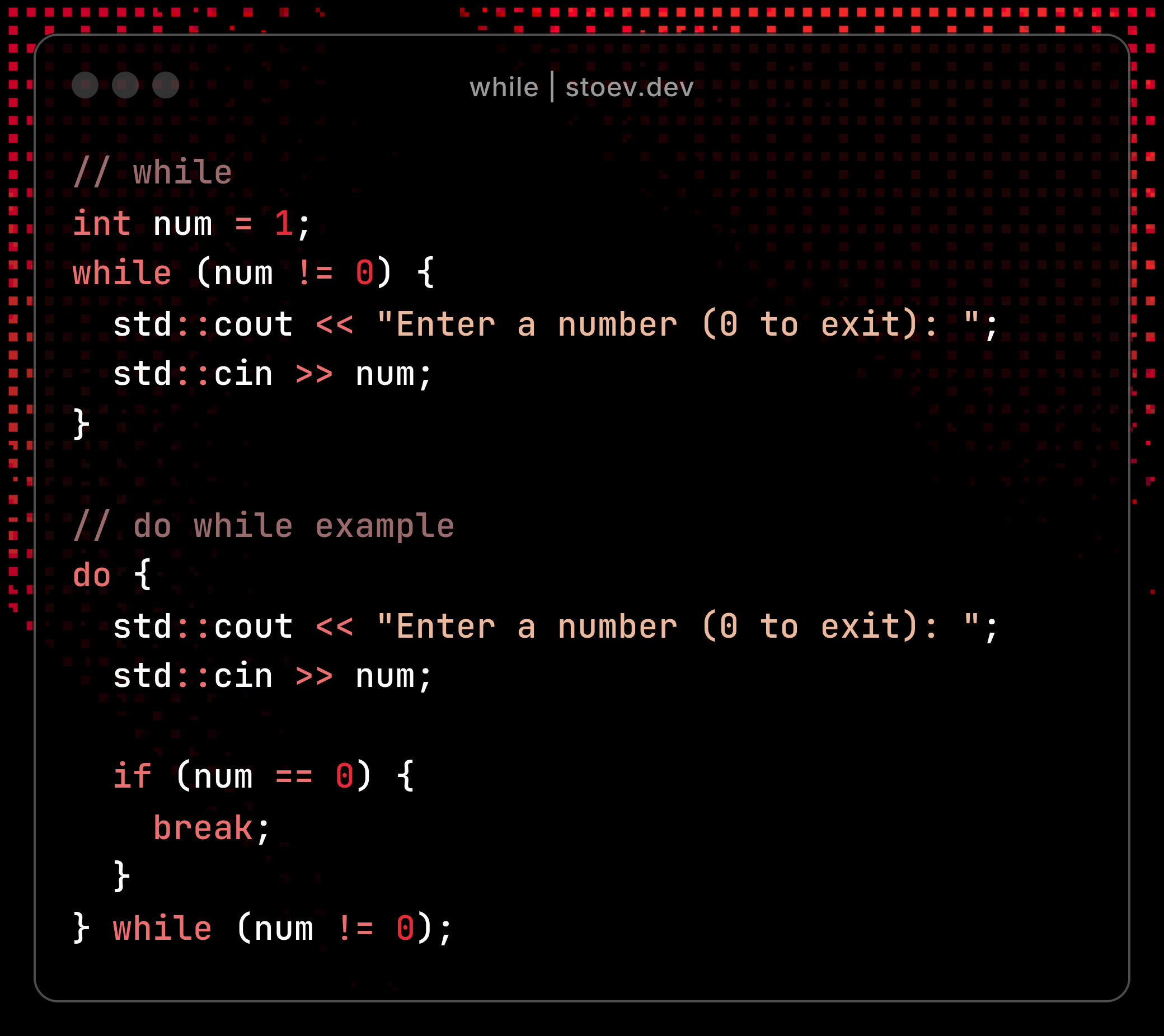
This is a simple program. It reads the numbers until 0 is entered.
- Precondition: The condition is checked before each iteration, so the loop may not execute if the condition is initially false.
- Initialization: Must occur before the loop to avoid undefined behavior.
The do while example is a little different. It guarantees at least one execution, checking the condition after each iteration.
- Use case: Ideal for menus or input validation where at least one attempt is required.
- Semicolon: Note the required ; after the while condition.
Control statements:
- break: Exits the innermost loop immediately.
- continue: Skips the rest of the current iteration, proceeding to the next.
Conditions
Conditional statements control program flow by executing code based on boolean conditions. They are essential for decision-making logic.
If Statements
C++ offers if, if-else, else-if, switch and the conditional (ternarry) operator. Conditions rely on relational (< , > , == , etc) and logical (&& , | | , !) operators.
- Condition: Must evaluate to bool. Non-boolean values (e.g. int) are implicitly converted (0 = false, non-zero = true)
- Short-circuit evaluation: Logical operators like && and | | evaluate only as far as needed (e.g. false && X skips X)
- Dangling Else: Ensure else aligns with the intended if using braces to avoid ambiguity.
Switch Statement
The switch statement handles multiple values efficiently, often cleaner than multiple if-else chains. It is a convenient way of selecting among fixed alternatives.
- Expression: Must evaluate to an integer or enumeration type (int, char). Floating points or string types are not allowed.
- Break: Prevents fall-through (executing subsequent cases). Omitting break is intentional in some cases but often a bug.
- Default: Optional, handles unmatched cases.
- Scope: Variables declared in a case are shared unless enclosed in braces { }.
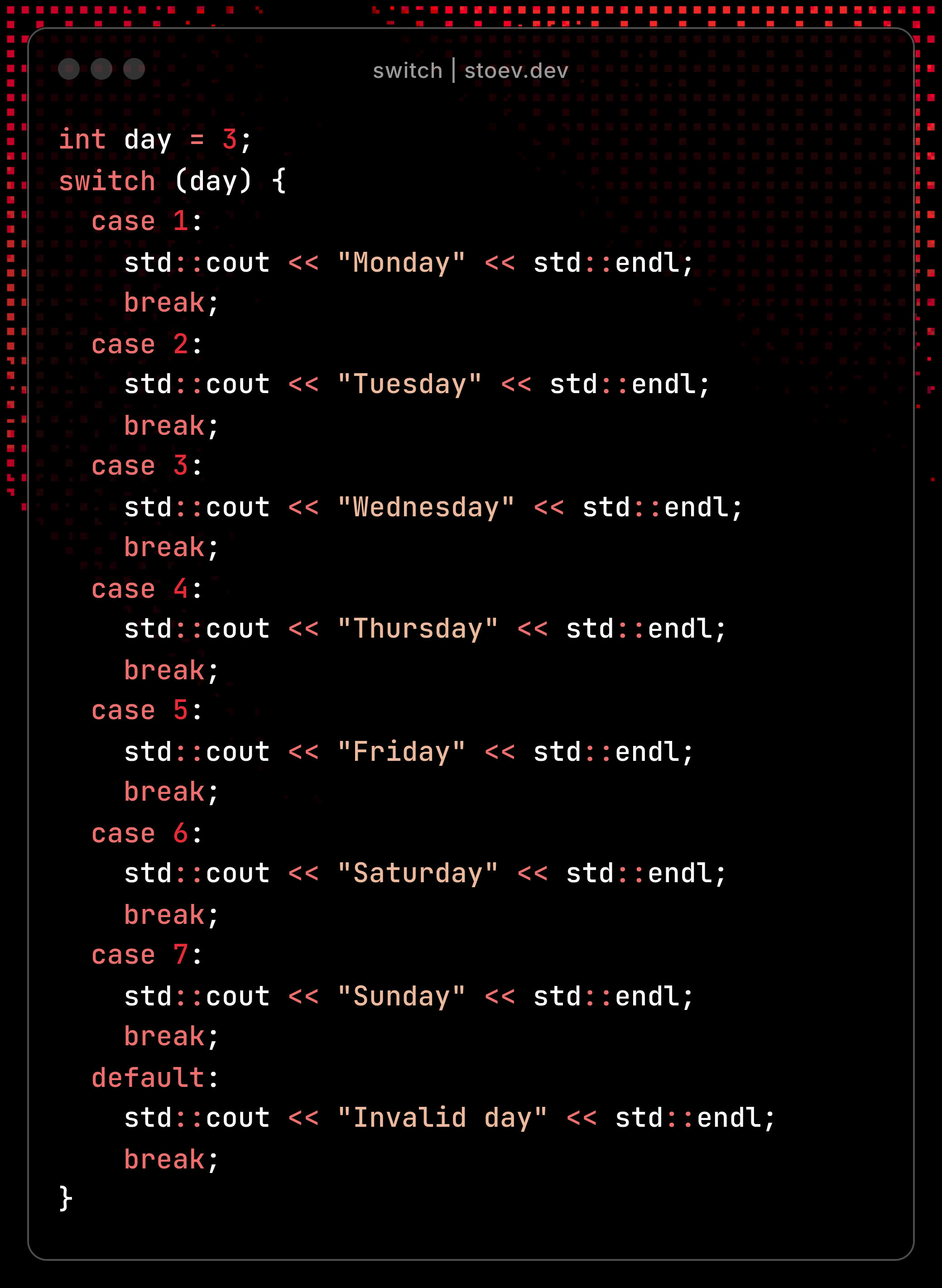
Conditional (Ternary) Operator
This is a concise alternative if-else for simple assignments. Syntax:
variable = (condition) ? valueIfTrue : valueIfFalse;
Use Case: Best for simple, single-expression decisions. Avoid nesting for readability.
Functions
Functions encapsulate reusable code, improving modularity and maintainability. They consist of a return type, name, parameters and a body. A function can’t be called unless it has been declared.
Functions allow code to be written once and called multiple times with different inputs. They can:
- Return a value (e.g. int) or nothing (void).
- Accept parameters or none.
- Be defined before or after main().
Invoke a function by name with arguments (if any).
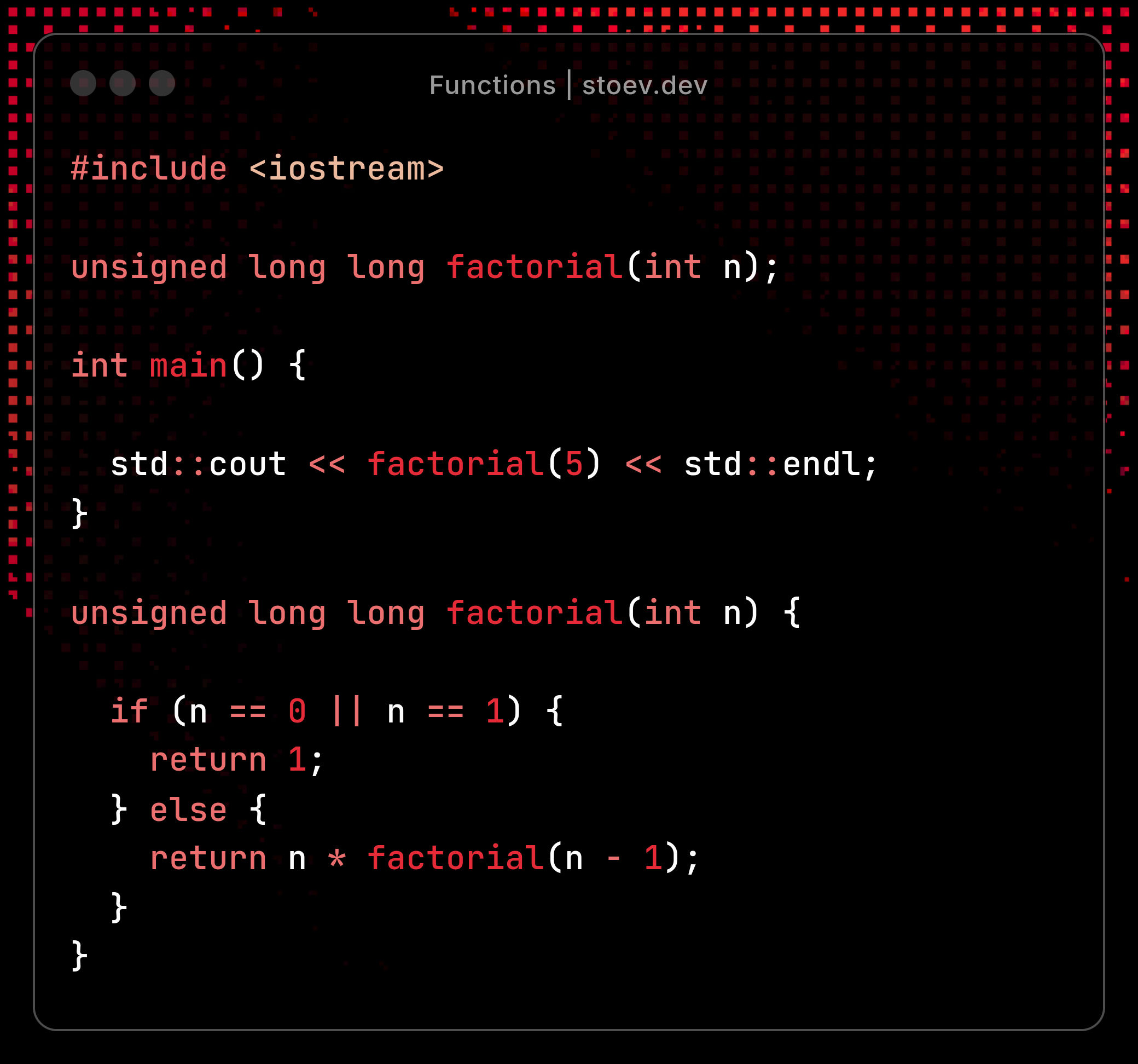
- Arguments: Passed by value (copy) or reference (address).
- Return: If non-void, the function must return a value matching the return type.
A variable defined in a function is commonly referred to as a local variable. If a variable is defined as static, the statically allocated object will be used to represent that variable in all calls of the function. Only the first time, it will be initialized.
Parameters can have default values, used if no argument is provided. Defaults must be specified from right to left. Default parameters must be in the declaration and not in the definition (if separate).
Function overloading
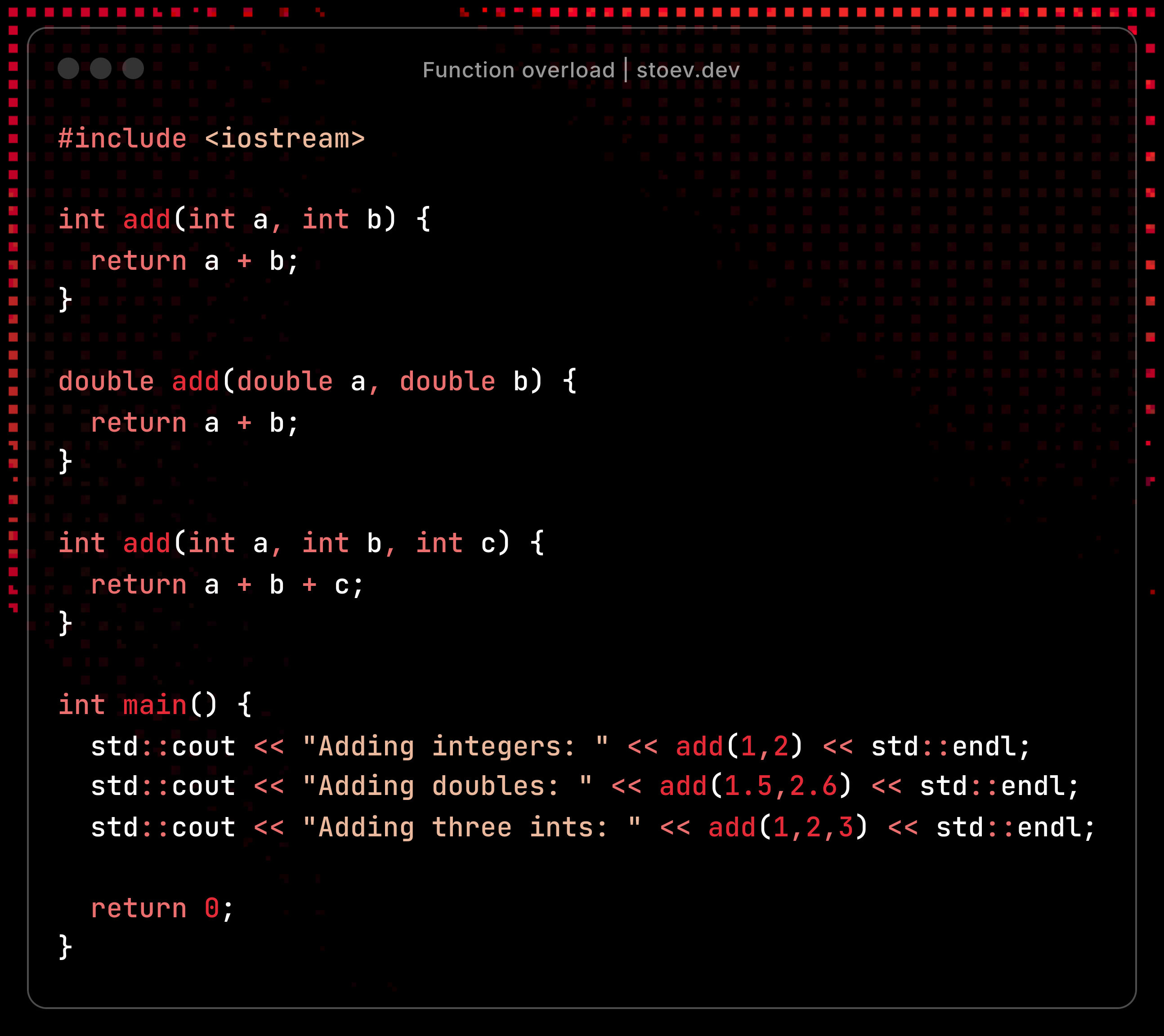
C++ supports multiple functions with the same name, but different parameter lists. The compiler selects the correct function based on the arguments.
The main function cannot be overloaded!
Overloading functions must differ in their arguments, not in their return type.
- Overload Resolution: The compiler matches arguments to signatures, considering implicit conversions. Ambiguities cause errors.
- Const Overloading: Functions can differ by parameter const-ness. (e.g. void print(const int&) vs void print(int&)).
- Default parameters can conflict with overloaded functions.
Pass by Value vs Pass by Reference
- Pass by Value: Copies the argument, leaving the original unchanged.
void increment(int x) {x++;} // No effect on caller.
- Pass by Reference: Passes the argument’s address, allowing modification.
void increment(int& x) {x++;} // Modifies caller’s variable.
- Const reference: Use const T& to pass large objects efficiently without copying, preventing modification. We can work with the referenced object, but we get errors if we try to modify it.
void print(const string& s) { cout << s << endl; }
- Performance: Reference passing avoids copying for large types (e.g. string, vector). Value passing is safer, but costlier for large objects.
Conclusion
This article explored C++’s variables, loops, conditions and functions. To master any of them, practice with the provided examples, experiment with variations. C++’s power lies in its flexibility and control - use it wisely to build reliable software.